Dokan API callbacks interface. More...
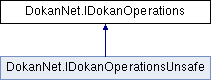
Public Member Functions | |
NtStatus | CreateFile (string fileName, FileAccess access, FileShare share, FileMode mode, FileOptions options, FileAttributes attributes, IDokanFileInfo info) |
CreateFile is called each time a request is made on a file system object. More... | |
void | Cleanup (string fileName, IDokanFileInfo info) |
Receipt of this request indicates that the last handle for a file object that is associated with the target device object has been closed (but, due to outstanding I/O requests, might not have been released). More... | |
void | CloseFile (string fileName, IDokanFileInfo info) |
CloseFile is called at the end of the life of the context. More... | |
NtStatus | ReadFile (string fileName, byte[] buffer, out int bytesRead, long offset, IDokanFileInfo info) |
ReadFile callback on the file previously opened in CreateFile. It can be called by different thread at the same time, therefor the read has to be thread safe. More... | |
NtStatus | WriteFile (string fileName, byte[] buffer, out int bytesWritten, long offset, IDokanFileInfo info) |
WriteFile callback on the file previously opened in CreateFile It can be called by different thread at the same time, therefor the write/context has to be thread safe. More... | |
NtStatus | FlushFileBuffers (string fileName, IDokanFileInfo info) |
Clears buffers for this context and causes any buffered data to be written to the file. More... | |
NtStatus | GetFileInformation (string fileName, out FileInformation fileInfo, IDokanFileInfo info) |
Get specific informations on a file. More... | |
NtStatus | FindFiles (string fileName, out IList< FileInformation > files, IDokanFileInfo info) |
List all files in the path requested More... | |
NtStatus | FindFilesWithPattern (string fileName, string searchPattern, out IList< FileInformation > files, IDokanFileInfo info) |
Same as FindFiles but with a search pattern to filter the result. More... | |
NtStatus | SetFileAttributes (string fileName, FileAttributes attributes, IDokanFileInfo info) |
Set file attributes on a specific file. More... | |
NtStatus | SetFileTime (string fileName, DateTime?creationTime, DateTime?lastAccessTime, DateTime?lastWriteTime, IDokanFileInfo info) |
Set file times on a specific file. If DateTime is null , this should not be updated. More... | |
NtStatus | DeleteFile (string fileName, IDokanFileInfo info) |
Check if it is possible to delete a file. More... | |
NtStatus | DeleteDirectory (string fileName, IDokanFileInfo info) |
Check if it is possible to delete a directory. More... | |
NtStatus | MoveFile (string oldName, string newName, bool replace, IDokanFileInfo info) |
Move a file or directory to a new location. More... | |
NtStatus | SetEndOfFile (string fileName, long length, IDokanFileInfo info) |
SetEndOfFile is used to truncate or extend a file (physical file size). More... | |
NtStatus | SetAllocationSize (string fileName, long length, IDokanFileInfo info) |
SetAllocationSize is used to truncate or extend a file. More... | |
NtStatus | LockFile (string fileName, long offset, long length, IDokanFileInfo info) |
Lock file at a specific offset and data length. This is only used if DokanOptions.UserModeLock is enabled. More... | |
NtStatus | UnlockFile (string fileName, long offset, long length, IDokanFileInfo info) |
Unlock file at a specific offset and data length. This is only used if DokanOptions.UserModeLock is enabled. More... | |
NtStatus | GetDiskFreeSpace (out long freeBytesAvailable, out long totalNumberOfBytes, out long totalNumberOfFreeBytes, IDokanFileInfo info) |
Retrieves information about the amount of space that is available on a disk volume, which is the total amount of space, the total amount of free space, and the total amount of free space available to the user that is associated with the calling thread. More... | |
NtStatus | GetVolumeInformation (out string volumeLabel, out FileSystemFeatures features, out string fileSystemName, out uint maximumComponentLength, IDokanFileInfo info) |
Retrieves information about the file system and volume associated with the specified root directory. More... | |
NtStatus | GetFileSecurity (string fileName, out FileSystemSecurity security, AccessControlSections sections, IDokanFileInfo info) |
Get specified information about the security of a file or directory. More... | |
NtStatus | SetFileSecurity (string fileName, FileSystemSecurity security, AccessControlSections sections, IDokanFileInfo info) |
Sets the security of a file or directory object. More... | |
NtStatus | Mounted (string mountPoint, IDokanFileInfo info) |
Is called when Dokan succeed to mount the volume. More... | |
NtStatus | Unmounted (IDokanFileInfo info) |
Is called when Dokan is unmounting the volume. More... | |
NtStatus | FindStreams (string fileName, out IList< FileInformation > streams, IDokanFileInfo info) |
Retrieve all NTFS Streams informations on the file. This is only called if DokanOptions.AltStream is enabled. More... | |
Detailed Description
Dokan API callbacks interface.
A interface of callbacks that describe all Dokan API operation that will be called when Windows access to the file system.
All this callbacks can return NtStatus.NotImplemented if you dont want to support one of them. Be aware that returning such value to important callbacks such CreateFile/ReadFile/... would make the filesystem not working or unstable.
This is the same struct as DOKAN_OPERATIONS
(dokan.h) in the C version of Dokan.
Member Function Documentation
void DokanNet.IDokanOperations.Cleanup | ( | string | fileName, |
IDokanFileInfo | info | ||
) |
Receipt of this request indicates that the last handle for a file object that is associated with the target device object has been closed (but, due to outstanding I/O requests, might not have been released).
Cleanup is requested before CloseFile is called.
When IDokanFileInfo.DeleteOnClose is true
, you must delete the file in Cleanup. Refer to DeleteFile and DeleteDirectory for explanation.
- Parameters
-
fileName File path requested by the Kernel on the FileSystem. info An IDokanFileInfo with information about the file or directory.
- See also
- DeleteFile, DeleteDirectory, CloseFile
void DokanNet.IDokanOperations.CloseFile | ( | string | fileName, |
IDokanFileInfo | info | ||
) |
CloseFile is called at the end of the life of the context.
Receipt of this request indicates that the last handle of the file object that is associated with the target device object has been closed and released. All outstanding I/O requests have been completed or canceled.
CloseFile is requested after Cleanup is called.
Remainings in IDokanFileInfo.Context has to be cleared before return.
- Parameters
-
fileName File path requested by the Kernel on the FileSystem. info An IDokanFileInfo with information about the file or directory.
- See also
- Cleanup
NtStatus DokanNet.IDokanOperations.CreateFile | ( | string | fileName, |
FileAccess | access, | ||
FileShare | share, | ||
FileMode | mode, | ||
FileOptions | options, | ||
FileAttributes | attributes, | ||
IDokanFileInfo | info | ||
) |
CreateFile is called each time a request is made on a file system object.
In case mode is FileMode.OpenOrCreate
and FileMode.Create
and CreateFile are successfully opening a already existing file, you have to return DokanResult.AlreadyExists instead of NtStatus.Success.
If the file is a directory, CreateFile is also called. In this case, CreateFile should return NtStatus.Success when that directory can be opened and IDokanFileInfo.IsDirectory must be set to true
. On the other hand, if IDokanFileInfo.IsDirectory is set to true
but the path target a file, you need to return DokanResult.NotADirectory
IDokanFileInfo.Context can be used to store data (like FileStream
) that can be retrieved in all other request related to the context.
- Parameters
-
fileName File path requested by the Kernel on the FileSystem. access A FileAccess with permissions for file or directory. share Type of share access to other threads, which is specified as FileShare.None or any combination of FileShare. Device and intermediate drivers usually set ShareAccess to zero, which gives the caller exclusive access to the open file. mode Specifies how the operating system should open a file. See FileMode Enumeration (MSDN). options Represents advanced options for creating a FileStream object. See FileOptions Enumeration (MSDN). attributes Provides attributes for files and directories. See FileAttributes Enumeration (MSDN>. info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
- See also
- See ZwCreateFile (MSDN) for more information about the parameters of this callback.
NtStatus DokanNet.IDokanOperations.DeleteDirectory | ( | string | fileName, |
IDokanFileInfo | info | ||
) |
Check if it is possible to delete a directory.
You should NOT delete the file in DeleteDirectory, but instead you must only check whether you can delete the file or not, and return NtStatus.Success (when you can delete it) or appropriate error codes such as NtStatus.AccessDenied, NtStatus.ObjectPathNotFound, NtStatus.ObjectNameNotFound.
DeleteFile will also be called with IDokanFileInfo.DeleteOnClose set to false
to notify the driver when the file is no longer requested to be deleted.
When you return NtStatus.Success, you get a Cleanup call afterwards with IDokanFileInfo.DeleteOnClose set to true
and only then you have to actually delete the file being closed.
- Parameters
-
fileName File path requested by the Kernel on the FileSystem. info An IDokanFileInfo with information about the file or directory.
- Returns
- Return DokanResult.Success if file can be delete or NtStatus appropriate.
- See also
- DeleteFile, Cleanup
NtStatus DokanNet.IDokanOperations.DeleteFile | ( | string | fileName, |
IDokanFileInfo | info | ||
) |
Check if it is possible to delete a file.
You should NOT delete the file in DeleteFile, but instead you must only check whether you can delete the file or not, and return NtStatus.Success (when you can delete it) or appropriate error codes such as NtStatus.AccessDenied, NtStatus.ObjectNameNotFound.
DeleteFile will also be called with IDokanFileInfo.DeleteOnClose set to false
to notify the driver when the file is no longer requested to be deleted.
When you return NtStatus.Success, you get a Cleanup call afterwards with IDokanFileInfo.DeleteOnClose set to true
and only then you have to actually delete the file being closed.
- Parameters
-
fileName File path requested by the Kernel on the FileSystem. info An IDokanFileInfo with information about the file or directory.
- Returns
- Return DokanResult.Success if file can be delete or NtStatus appropriate.
- See also
- DeleteDirectory, Cleanup
NtStatus DokanNet.IDokanOperations.FindFiles | ( | string | fileName, |
out IList< FileInformation > | files, | ||
IDokanFileInfo | info | ||
) |
List all files in the path requested
FindFilesWithPattern is checking first. If it is not implemented or returns NtStatus.NotImplemented, then FindFiles is called.
- Parameters
-
fileName File path requested by the Kernel on the FileSystem. files A list of FileInformation to return. info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
- See also
- FindFilesWithPattern
NtStatus DokanNet.IDokanOperations.FindFilesWithPattern | ( | string | fileName, |
string | searchPattern, | ||
out IList< FileInformation > | files, | ||
IDokanFileInfo | info | ||
) |
Same as FindFiles but with a search pattern to filter the result.
- Parameters
-
fileName Path requested by the Kernel on the FileSystem. searchPattern Search pattern files A list of FileInformation to return. info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
- See also
- FindFiles
NtStatus DokanNet.IDokanOperations.FindStreams | ( | string | fileName, |
out IList< FileInformation > | streams, | ||
IDokanFileInfo | info | ||
) |
Retrieve all NTFS Streams informations on the file. This is only called if DokanOptions.AltStream is enabled.
For files, the first item in streams is information about the default data stream "::$DATA"
.
- Since
- Supported since version 0.8.0. You must specify the Native.DOKAN_OPTIONS.Version during DokanInstance.DokanInstance.
- Parameters
-
fileName File path requested by the Kernel on the FileSystem. streams List of FileInformation for each streams present on the file. info An IDokanFileInfo with information about the file or directory.
- Returns
- Return NtStatus or DokanResult appropriate to the request result.
NtStatus DokanNet.IDokanOperations.FlushFileBuffers | ( | string | fileName, |
IDokanFileInfo | info | ||
) |
Clears buffers for this context and causes any buffered data to be written to the file.
- Parameters
-
fileName File path requested by the Kernel on the FileSystem. info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
NtStatus DokanNet.IDokanOperations.GetDiskFreeSpace | ( | out long | freeBytesAvailable, |
out long | totalNumberOfBytes, | ||
out long | totalNumberOfFreeBytes, | ||
IDokanFileInfo | info | ||
) |
Retrieves information about the amount of space that is available on a disk volume, which is the total amount of space, the total amount of free space, and the total amount of free space available to the user that is associated with the calling thread.
Neither GetDiskFreeSpace nor GetVolumeInformation save the IDokanFileInfo.Context. Before these methods are called, CreateFile may not be called. (ditto CloseFile and Cleanup).
- Parameters
-
freeBytesAvailable Amount of available space. totalNumberOfBytes Total size of storage space. totalNumberOfFreeBytes Amount of free space. info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
- See also
- GetDiskFreeSpaceEx function (MSDN)
- See also
- GetVolumeInformation
NtStatus DokanNet.IDokanOperations.GetFileInformation | ( | string | fileName, |
out FileInformation | fileInfo, | ||
IDokanFileInfo | info | ||
) |
Get specific informations on a file.
- Parameters
-
fileName File path requested by the Kernel on the FileSystem. fileInfo FileInformation struct to fill info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
NtStatus DokanNet.IDokanOperations.GetFileSecurity | ( | string | fileName, |
out FileSystemSecurity | security, | ||
AccessControlSections | sections, | ||
IDokanFileInfo | info | ||
) |
Get specified information about the security of a file or directory.
If NtStatus.NotImplemented is returned, dokan library will handle the request by building a sddl of the current process user with authenticate user rights for context menu.
- Since
- Supported since version 0.6.0. You must specify the Native.DOKAN_OPTIONS.Version during DokanInstance.DokanInstance.
- Parameters
-
fileName File or directory name. security A FileSystemSecurity with security information to return. sections A AccessControlSections with access sections to return. info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
NtStatus DokanNet.IDokanOperations.GetVolumeInformation | ( | out string | volumeLabel, |
out FileSystemFeatures | features, | ||
out string | fileSystemName, | ||
out uint | maximumComponentLength, | ||
IDokanFileInfo | info | ||
) |
Retrieves information about the file system and volume associated with the specified root directory.
Neither GetVolumeInformation nor GetDiskFreeSpace save the IDokanFileInfo.Context. Before these methods are called, CreateFile may not be called. (ditto CloseFile and Cleanup).
FileSystemFeatures.ReadOnlyVolume is automatically added to the features if DokanOptions.WriteProtection was specified when the volume was mounted.
If NtStatus.NotImplemented is returned, the Dokan kernel driver use following settings by default:
Parameter | Default value |
---|---|
rawVolumeNameBuffer | "DOKAN" |
rawVolumeSerialNumber | 0x19831116 |
rawMaximumComponentLength | 256 |
rawFileSystemFlags | CaseSensitiveSearch || CasePreservedNames || SupportsRemoteStorage || UnicodeOnDisk |
rawFileSystemNameBuffer | "NTFS" |
- Parameters
-
volumeLabel Volume name features FileSystemFeatures with features enabled on the volume. fileSystemName The name of the specified volume. maximumComponentLength File name component that the specified file system supports. info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
NtStatus DokanNet.IDokanOperations.LockFile | ( | string | fileName, |
long | offset, | ||
long | length, | ||
IDokanFileInfo | info | ||
) |
Lock file at a specific offset and data length. This is only used if DokanOptions.UserModeLock is enabled.
- Parameters
-
fileName File path requested by the Kernel on the FileSystem. offset Offset from where the lock has to be proceed. length Data length to lock. info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
- See also
- UnlockFile
NtStatus DokanNet.IDokanOperations.Mounted | ( | string | mountPoint, |
IDokanFileInfo | info | ||
) |
Is called when Dokan succeed to mount the volume.
If DokanOptions.MountManager is enabled and the drive letter requested is busy, the mountPoint can contain a different drive letter that the mount manager assigned us.
- Parameters
-
mountPoint The mount point assign to the instance. info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
NtStatus DokanNet.IDokanOperations.MoveFile | ( | string | oldName, |
string | newName, | ||
bool | replace, | ||
IDokanFileInfo | info | ||
) |
Move a file or directory to a new location.
- Parameters
-
oldName Path to the file to move. newName Path to the new location for the file. replace If the file should be replaced if it already exist a file with path newName . info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
NtStatus DokanNet.IDokanOperations.ReadFile | ( | string | fileName, |
byte[] | buffer, | ||
out int | bytesRead, | ||
long | offset, | ||
IDokanFileInfo | info | ||
) |
ReadFile callback on the file previously opened in CreateFile. It can be called by different thread at the same time, therefor the read has to be thread safe.
- Parameters
-
fileName File path requested by the Kernel on the FileSystem. buffer Read buffer that has to be fill with the read result. The buffer size depend of the read size requested by the kernel. bytesRead Total number of bytes that has been read. offset Offset from where the read has to be proceed. info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
- See also
- WriteFile
NtStatus DokanNet.IDokanOperations.SetAllocationSize | ( | string | fileName, |
long | length, | ||
IDokanFileInfo | info | ||
) |
SetAllocationSize is used to truncate or extend a file.
- Parameters
-
fileName File path requested by the Kernel on the FileSystem. length File length to set info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
NtStatus DokanNet.IDokanOperations.SetEndOfFile | ( | string | fileName, |
long | length, | ||
IDokanFileInfo | info | ||
) |
SetEndOfFile is used to truncate or extend a file (physical file size).
- Parameters
-
fileName File path requested by the Kernel on the FileSystem. length File length to set info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
NtStatus DokanNet.IDokanOperations.SetFileAttributes | ( | string | fileName, |
FileAttributes | attributes, | ||
IDokanFileInfo | info | ||
) |
Set file attributes on a specific file.
SetFileAttributes and SetFileTime are called only if both of them are implemented.
- Parameters
-
fileName File path requested by the Kernel on the FileSystem. attributes FileAttributes to set on file info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
NtStatus DokanNet.IDokanOperations.SetFileSecurity | ( | string | fileName, |
FileSystemSecurity | security, | ||
AccessControlSections | sections, | ||
IDokanFileInfo | info | ||
) |
Sets the security of a file or directory object.
- Since
- Supported since version 0.6.0. You must specify the Native.DOKAN_OPTIONS.Version during DokanInstance.DokanInstance.
- Parameters
-
fileName File path requested by the Kernel on the FileSystem. security A FileSystemSecurity with security information to set. sections A AccessControlSections with access sections on which. info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
NtStatus DokanNet.IDokanOperations.SetFileTime | ( | string | fileName, |
DateTime? | creationTime, | ||
DateTime? | lastAccessTime, | ||
DateTime? | lastWriteTime, | ||
IDokanFileInfo | info | ||
) |
Set file times on a specific file. If DateTime is null
, this should not be updated.
SetFileAttributes and SetFileTime are called only if both of them are implemented.
- Parameters
-
fileName File or directory name. creationTime DateTime when the file was created. lastAccessTime DateTime when the file was last accessed. lastWriteTime DateTime when the file was last written to. info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
NtStatus DokanNet.IDokanOperations.UnlockFile | ( | string | fileName, |
long | offset, | ||
long | length, | ||
IDokanFileInfo | info | ||
) |
Unlock file at a specific offset and data length. This is only used if DokanOptions.UserModeLock is enabled.
- Parameters
-
fileName File path requested by the Kernel on the FileSystem. offset Offset from where the unlock has to be proceed. length Data length to lock. info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
- See also
- LockFile
NtStatus DokanNet.IDokanOperations.Unmounted | ( | IDokanFileInfo | info | ) |
Is called when Dokan is unmounting the volume.
- Parameters
-
info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
- See also
- Mounted
NtStatus DokanNet.IDokanOperations.WriteFile | ( | string | fileName, |
byte[] | buffer, | ||
out int | bytesWritten, | ||
long | offset, | ||
IDokanFileInfo | info | ||
) |
WriteFile callback on the file previously opened in CreateFile It can be called by different thread at the same time, therefor the write/context has to be thread safe.
- Parameters
-
fileName File path requested by the Kernel on the FileSystem. buffer Data that has to be written. bytesWritten Total number of bytes that has been write. offset Offset from where the write has to be proceed. info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
- See also
- ReadFile
The documentation for this interface was generated from the following file:
- IDokanOperations.cs