This is a sub-interface of IDokanOperations that can optionally be implemented to get access to the raw, unmanaged buffers for ReadFile() and WriteFile() for performance optimization. Marshalling the unmanaged buffers to and from byte[] arrays for every call of these APIs incurs an extra copy that can be avoided by reading from or writing directly to the unmanaged buffers. More...
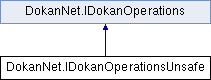
Public Member Functions | |
NtStatus | ReadFile (string fileName, IntPtr buffer, uint bufferLength, out int bytesRead, long offset, IDokanFileInfo info) |
ReadFile callback on the file previously opened in IDokanOperations.CreateFile. It can be called by different thread at the same time, therefore the read has to be thread safe. More... | |
NtStatus | WriteFile (string fileName, IntPtr buffer, uint bufferLength, out int bytesWritten, long offset, IDokanFileInfo info) |
WriteFile callback on the file previously opened in IDokanOperations.CreateFile It can be called by different thread at the same time, therefore the write/context has to be thread safe. More... | |
![]() | |
NtStatus | CreateFile (string fileName, FileAccess access, FileShare share, FileMode mode, FileOptions options, FileAttributes attributes, IDokanFileInfo info) |
CreateFile is called each time a request is made on a file system object. More... | |
void | Cleanup (string fileName, IDokanFileInfo info) |
Receipt of this request indicates that the last handle for a file object that is associated with the target device object has been closed (but, due to outstanding I/O requests, might not have been released). More... | |
void | CloseFile (string fileName, IDokanFileInfo info) |
CloseFile is called at the end of the life of the context. More... | |
NtStatus | ReadFile (string fileName, byte[] buffer, out int bytesRead, long offset, IDokanFileInfo info) |
ReadFile callback on the file previously opened in CreateFile. It can be called by different thread at the same time, therefor the read has to be thread safe. More... | |
NtStatus | WriteFile (string fileName, byte[] buffer, out int bytesWritten, long offset, IDokanFileInfo info) |
WriteFile callback on the file previously opened in CreateFile It can be called by different thread at the same time, therefor the write/context has to be thread safe. More... | |
NtStatus | FlushFileBuffers (string fileName, IDokanFileInfo info) |
Clears buffers for this context and causes any buffered data to be written to the file. More... | |
NtStatus | GetFileInformation (string fileName, out FileInformation fileInfo, IDokanFileInfo info) |
Get specific informations on a file. More... | |
NtStatus | FindFiles (string fileName, out IList< FileInformation > files, IDokanFileInfo info) |
List all files in the path requested More... | |
NtStatus | FindFilesWithPattern (string fileName, string searchPattern, out IList< FileInformation > files, IDokanFileInfo info) |
Same as FindFiles but with a search pattern to filter the result. More... | |
NtStatus | SetFileAttributes (string fileName, FileAttributes attributes, IDokanFileInfo info) |
Set file attributes on a specific file. More... | |
NtStatus | SetFileTime (string fileName, DateTime?creationTime, DateTime?lastAccessTime, DateTime?lastWriteTime, IDokanFileInfo info) |
Set file times on a specific file. If DateTime is null , this should not be updated. More... | |
NtStatus | DeleteFile (string fileName, IDokanFileInfo info) |
Check if it is possible to delete a file. More... | |
NtStatus | DeleteDirectory (string fileName, IDokanFileInfo info) |
Check if it is possible to delete a directory. More... | |
NtStatus | MoveFile (string oldName, string newName, bool replace, IDokanFileInfo info) |
Move a file or directory to a new location. More... | |
NtStatus | SetEndOfFile (string fileName, long length, IDokanFileInfo info) |
SetEndOfFile is used to truncate or extend a file (physical file size). More... | |
NtStatus | SetAllocationSize (string fileName, long length, IDokanFileInfo info) |
SetAllocationSize is used to truncate or extend a file. More... | |
NtStatus | LockFile (string fileName, long offset, long length, IDokanFileInfo info) |
Lock file at a specific offset and data length. This is only used if DokanOptions.UserModeLock is enabled. More... | |
NtStatus | UnlockFile (string fileName, long offset, long length, IDokanFileInfo info) |
Unlock file at a specific offset and data length. This is only used if DokanOptions.UserModeLock is enabled. More... | |
NtStatus | GetDiskFreeSpace (out long freeBytesAvailable, out long totalNumberOfBytes, out long totalNumberOfFreeBytes, IDokanFileInfo info) |
Retrieves information about the amount of space that is available on a disk volume, which is the total amount of space, the total amount of free space, and the total amount of free space available to the user that is associated with the calling thread. More... | |
NtStatus | GetVolumeInformation (out string volumeLabel, out FileSystemFeatures features, out string fileSystemName, out uint maximumComponentLength, IDokanFileInfo info) |
Retrieves information about the file system and volume associated with the specified root directory. More... | |
NtStatus | GetFileSecurity (string fileName, out FileSystemSecurity?security, AccessControlSections sections, IDokanFileInfo info) |
Get specified information about the security of a file or directory. More... | |
NtStatus | SetFileSecurity (string fileName, FileSystemSecurity security, AccessControlSections sections, IDokanFileInfo info) |
Sets the security of a file or directory object. More... | |
NtStatus | Mounted (string mountPoint, IDokanFileInfo info) |
Is called when Dokan succeed to mount the volume. More... | |
NtStatus | Unmounted (IDokanFileInfo info) |
Is called when Dokan is unmounting the volume. More... | |
NtStatus | FindStreams (string fileName, out IList< FileInformation > streams, IDokanFileInfo info) |
Retrieve all NTFS Streams informations on the file. This is only called if DokanOptions.AltStream is enabled. More... | |
Detailed Description
This is a sub-interface of IDokanOperations that can optionally be implemented to get access to the raw, unmanaged buffers for ReadFile() and WriteFile() for performance optimization. Marshalling the unmanaged buffers to and from byte[] arrays for every call of these APIs incurs an extra copy that can be avoided by reading from or writing directly to the unmanaged buffers.
Implementation of this interface is optional. If it is implemented, the overloads of Read/WriteFile(IntPtr, length) will be called instead of Read/WriteFile(byte[]). The caller can fill or read from the unmanaged API with Marshal.Copy, Buffer.MemoryCopy or similar.
Member Function Documentation
NtStatus DokanNet.IDokanOperationsUnsafe.ReadFile | ( | string | fileName, |
IntPtr | buffer, | ||
uint | bufferLength, | ||
out int | bytesRead, | ||
long | offset, | ||
IDokanFileInfo | info | ||
) |
ReadFile callback on the file previously opened in IDokanOperations.CreateFile. It can be called by different thread at the same time, therefore the read has to be thread safe.
- Parameters
-
fileName File path requested by the Kernel on the FileSystem. buffer Read buffer that has to be fill with the read result. bufferLength The size of 'buffer' in bytes. The buffer size depends of the read size requested by the kernel. bytesRead Total number of bytes that has been read. offset Offset from where the read has to be proceed. info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
- See also
- WriteFile
NtStatus DokanNet.IDokanOperationsUnsafe.WriteFile | ( | string | fileName, |
IntPtr | buffer, | ||
uint | bufferLength, | ||
out int | bytesWritten, | ||
long | offset, | ||
IDokanFileInfo | info | ||
) |
WriteFile callback on the file previously opened in IDokanOperations.CreateFile It can be called by different thread at the same time, therefore the write/context has to be thread safe.
- Parameters
-
fileName File path requested by the Kernel on the FileSystem. buffer Data that has to be written. bufferLength The size of 'buffer' in bytes. bytesWritten Total number of bytes that has been write. offset Offset from where the write has to be proceed. info An IDokanFileInfo with information about the file or directory.
- Returns
- NtStatus or DokanResult appropriate to the request result.
- See also
- ReadFile
The documentation for this interface was generated from the following file:
- IDokanOperationsUnsafe.cs